Unreal Engine Programming - Tips
Display FPS Counter
Blueprintの例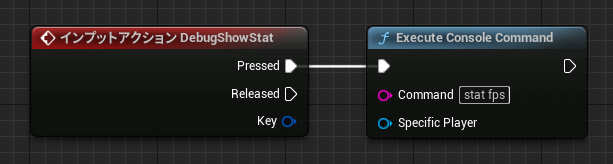
Display FPS Counter
C++版
数字キー5押下に割り当て 入力設定例: アクションマップで任意の入力値を設定する DebugShowStat:5 void ACPPCharacter::DebugShowStat(void) { UKismetSystemLibrary::ExecuteConsoleCommand(this, "stat fps"); UKismetSystemLibrary::ExecuteConsoleCommand(this, "t.maxfps 60"); // FPS制限 } in SetupPlayerInputComponent(class UInputComponent *InputComponent); InputComponent->BindAction("DebugShowStat", IE_Pressed, this, &ACharacter::DebugShowStat);
Run C++版と Blueprint版
// Remote Precedcure Call // RunCPP UFUNCTION(Server, Reliable) void ServerRun(); UFUNCTION(Server, Reliable) void ServerStopRunning); void APawnDev::StartSprinting() { ServerRun(); isRunPressed = true; GetCharacterMovement()->MaxWalkSpeed = RunSpeed; } void APawnDev::StopSprinting() { ServerStopRunning(); isRunPressed = false; GetCharacterMovement()->MaxWalkSpeed = DefaultMaxWalkSpeed; } void APawnDev::ServerRun_Implementation() { isRunPressed = true; GetCharacterMovement()->MaxWalkSpeed = RunSpeed; } void APawnDev::ServerStopRunning_Implementation() { isRunPressed = false; GetCharacterMovement()->MaxWalkSpeed = DefaultMaxWalkSpeed; }
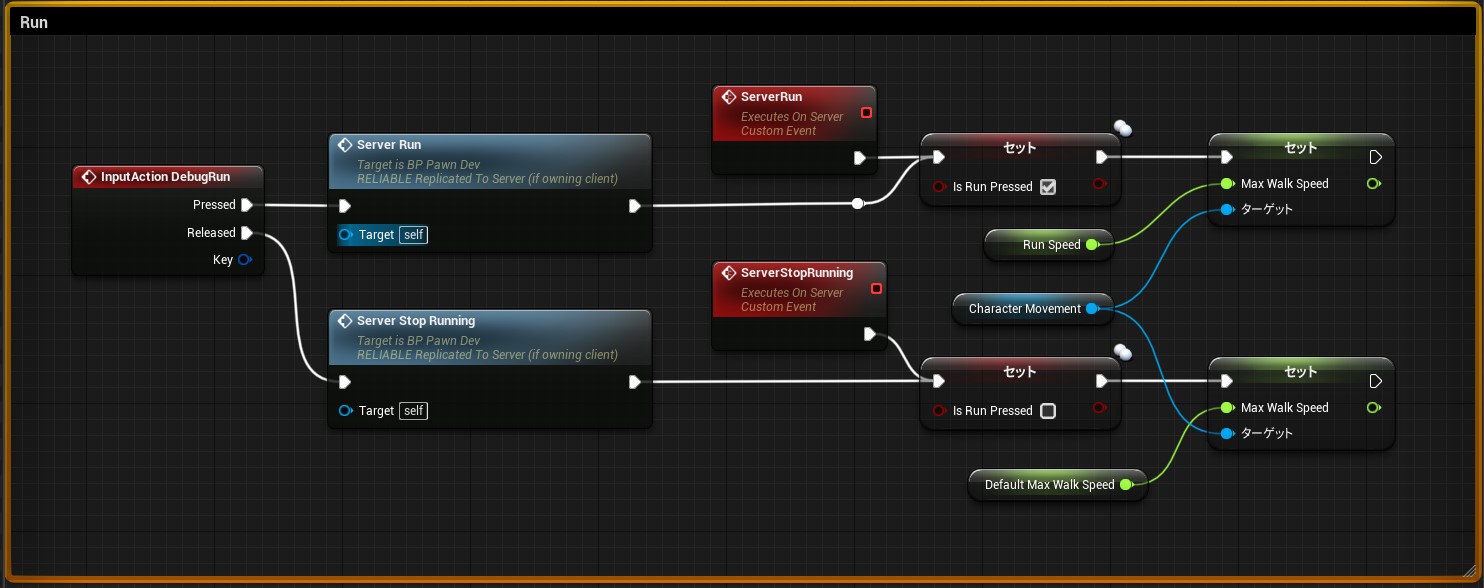
Line Trace - Blueprint
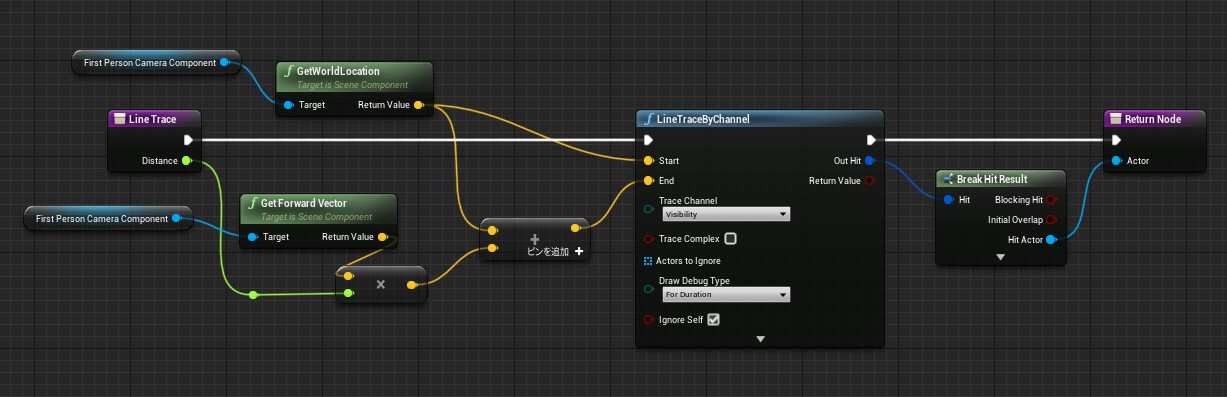
Line Trace Interact Door - C++ and Blueprint
Header: public: MyInput->BindAction("InteractDoor", IE_Pressed, this, &APawnDev::InteractDoor); UFUNCTION(BlueprintCallable) AActor* CCustomDoorLineTrace(float Distance); void InteractDoor(); UFUNCTION(BlueprintCallable, Category = "Door")) void ToggleDoor(AActor* Door); UFUNCTION(Server, Reliable) void ServerToggleDoor(); CPP: AActor* APawnDev::CCustomDoorLineTrace(float Distance) { FHitResult FrontHit; FCollisionQueryParams CollisionParams; CollisionParams.AddIgnoredActor(this); FVector Start; FVector End; //APlayerCameraManager* camManager = GetWorld()->GetFirstPlayerController()->PlayerCameraManager; //Start = camManager->GetCameraLocation(); Start = GetCameraLocation(); const FVector TraceOffset = GetFirstPersonCameraComponent()->GetForwardVector() * Distance; End = Start + TraceOffset; if (GetWorld()->LineTraceSingleByChannel(FrontHit, Start, End, ECC_Visibility, CollisionParams)) { GEngine->AddOnScreenDebugMessage(-1, 5.F, FColor::Green, TEXT("##### CCustomDoorLineTrace #####")); DrawDebugLine(GetWorld(), Start, FrontHit.Location, FColor::Red, true, 2.f); } return FrontHit.Actor.Get(); } void APawnDev::InteractDoor() { if (!HasAuthority()) { ServerToggleDoor(); } else { ToggleDoor(CCustomDoorLineTrace(200)); } } void APawnDev::ToggleDoor(AActor* InDoor) { ACustomDoor* Door = Cast(InDoor); if (Door) { Door->ToggleDoor(Door); } } void APawnDev::ServerToggleDoor_Implementation() { ToggleDoor(CCustomDoorLineTrace(200)); }
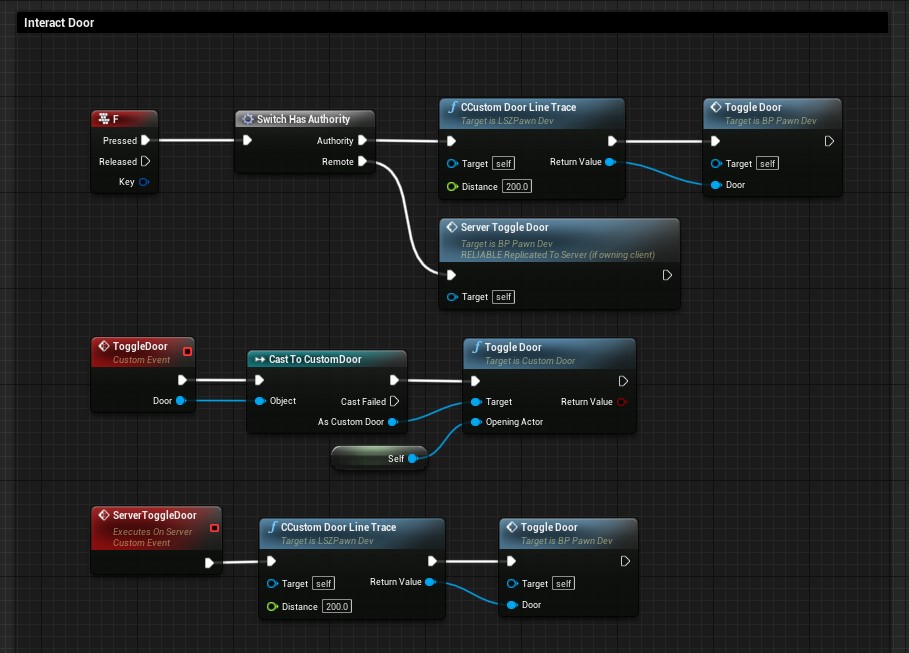
Sprint
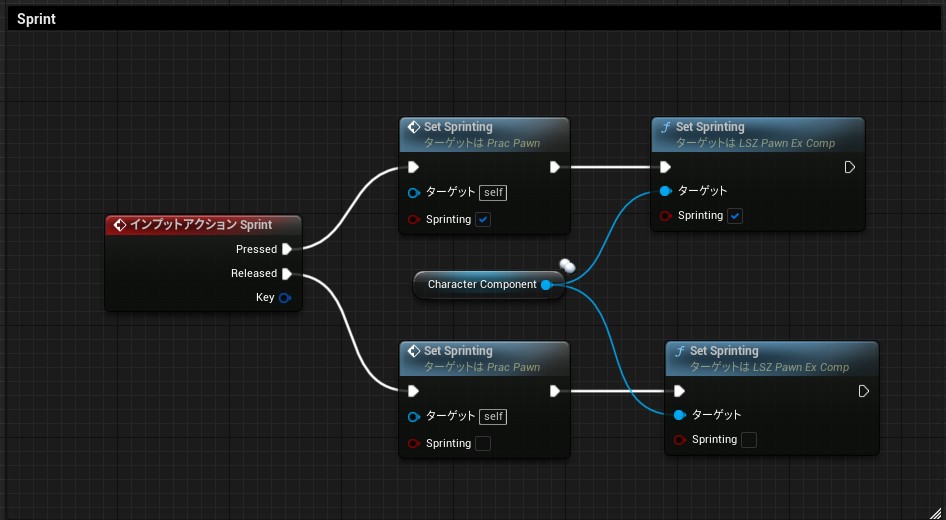
Set Sprinting
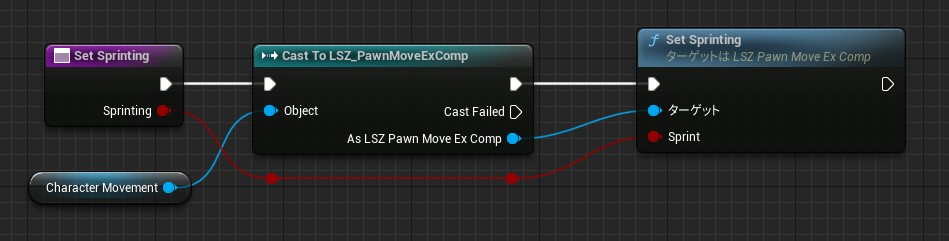
C++ Sprint - Set Sprinting
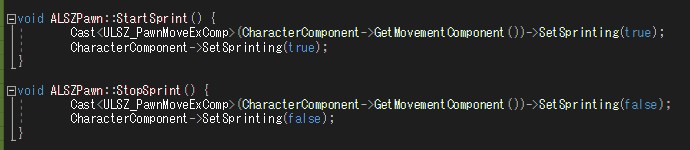
Jump
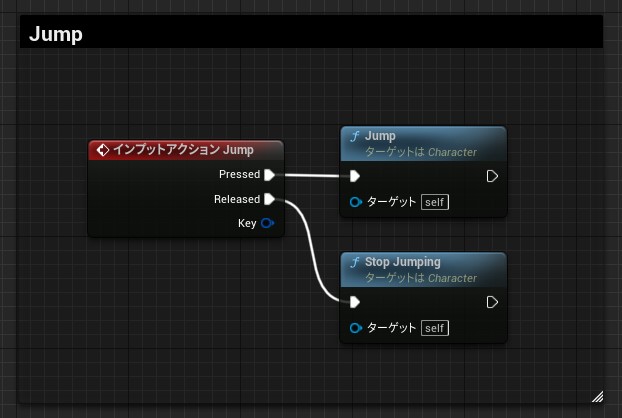
C++ Jump
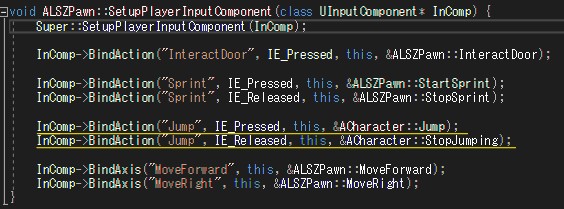