Unreal Engine Programming
Unreal Engine 5 - CPP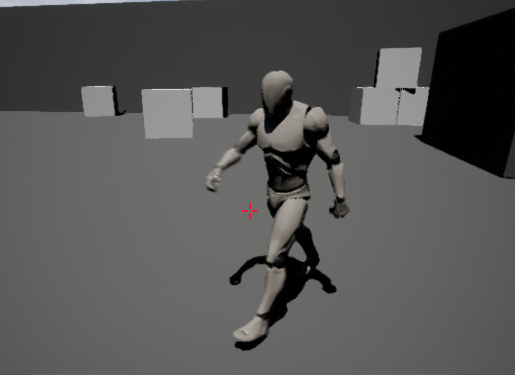
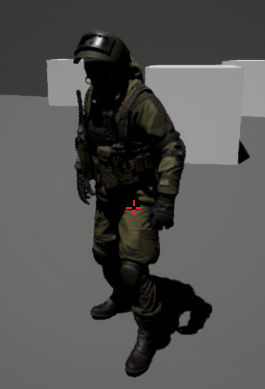
Unreal Engine(Common) - CPP
UHUDGameOverlay : UserWidget
HUDGameOverlay.h
HUDGameOverlay.h
#pragma once #include <MYFW/BasePlayerState.h> # Custom #include <Blueprint/UserWidget.h> # UE #include "HUDGameOverlay.generated.h" // Main overlay HUD UCLASS() class MYFW_API UHUDGameOverlay : public UUserWidget { GENERATED_BODY() public: }
ABasePlayerController : APlayerController
BasePlayerController.h
BasePlayerController.h
#pragma once #include <MYFW/Character/BaseCharacter.h> # Custom #include <MYFW/HUD/HUDGameOverlay.h> #include <GameFramework/PlayerController.h> #include "BasePlayerController.generated.h" UCLASS() class MYFW_API ABasePlayerController : public APlayerController { GENERATED_BODY() TSubclassOf<UHUDGameOverlay> MainOverlayClass; UPROPERTY() UHUDGameOverlay* MainOverlay; public: ABasePlayerController(); void BeginPlay() override; UFUNCTION(BlueprintCallable) void Spawn(); }
ABasePlayerController : APlayerController
BasePlayerController.cpp
BasePlayerController.cpp
#include <MYFW/BasePlayerController.h> #include <Net/UnrealNetwork.h> #include <MYFW/BaseWorldSettings.h> #include <MYFW/BaseGameMode.h> ABasePlayerController::ABasePlayerController() { static ConstructorHelpers::FObjectFinder<UObject>MainOverlayWClass(TEXT("WidgetBlueprint'/MYFW/HUD/GameOverlay.GameOverlay_C'")); MainOverlayClass = (UClass*)MainOverlayWClass.Object; } ABasePlayerController::BeginPlay() { GEngine->AddOnScreenDebugMessage(-1, 5.F, FColor::Blue, TEXT("BasePlayerController::BeginPlay")); Super::BeginPlay(); // Create MainOverlayWidget MainOverlay = CreateWidget<UHUDGameOverlay>(this, MainOverlayClass); if (MainOverlay) { MainOverlay->AddToViewport(10); } } void ABasePlayerController::Spawn() { if (GetNetMode() == NM_Client) { ServerSpawn(); return; } ABaseGameMode* GameMode = Cast<ABaseGameMode>(GetWorld()->GetAuthGameMode()); if (GameMode) { GameMode->PlayerSpawn(this); } } void ABasePlayerController::ServerSpawn_Implementation() { Spawn(); } bool ABasePlayerController::ServerSpawn_Validate() { return true; }
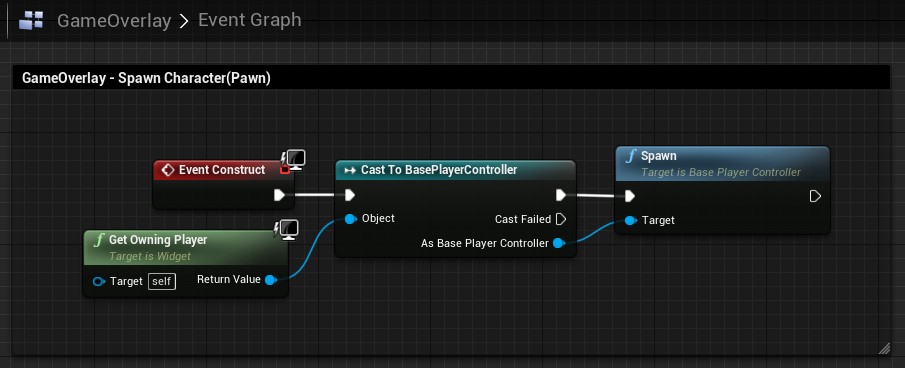