Unity3D - HUDFPS
4/27/2025 5:36:18 AM in Japan
参考URL wiki.unity3d.com FramesPerSecond
以下のC#コードを用意し
カメラオブジェクトにアタッチしてみます。
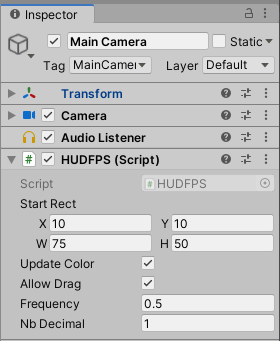
実行イメージ
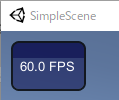
file:HUDFPS.cs
using UnityEngine; using System.Collections; public class HUDFPS : MonoBehaviour { // Attach this to any object to make a frame/second Indicator. // It calculates frames/second over each updateInterval. // so the display does not keep changing wildly. // We do this not by simple counting frames per interval. // but by accumlating FPS for each frame. // This way we end up with startRect overall FPS even // if the interval renders something like 5.5 frames. // The rect the window is initially display at. public Rect startRect = new Rect(10, 10, 35, 55); // Do you want the color change if the FPS gets low public bool updateColor = true; // Do you want to allow the dragging of the FPS window public bool allowDrag = true; // The update frequency of the fps public float frequency = 0.5F; // How many decimal do you want to display public int nbDecimal = 1; // FPS accumlated over the interval private float accum = 0F; // Frames down over the interval private int frames = 0; // The color of the GUI, depending of the FPS (R < 10, Y < 30.0 >= 30) private Color color = Color.white; // The fps formatted into a string private string sFPS = ""; // The style the text will be displayed based on defaultSkin.label. private GUIStyle style; // Start is called before the first frame update void Start() { StartCoroutine( FPS() ); } // Update is called once per frame void Update() { accum += Time.timeScale / Time.deltaTime; ++frames; } IEnumerator FPS() { // Infinite loop executed every "frequency" seconds. while( true ) { // Update the FPS float fps = accum / frames; sFPS = fps.ToString("f" + Mathf.Clamp(nbDecimal, 0, 10)); // Update the color color = (fps >= 30) ? Color.blue : ((fps > 10) ? Color.red : Color.yellow); accum = 0.0F; frames = 0; yield return new WaitForSeconds(frequency); } } private void OnGUI() { // Copy the default label skin, change the color and alignment if (style == null) { style = new GUIStyle(GUI.skin.label); style.normal.textColor = Color.white; style.alignment = TextAnchor.MiddleCenter; } GUI.color = updateColor ? color : Color.white; startRect = GUI.Window(0, startRect, DoMyWindow, ""); } void DoMyWindow(int windowID) { GUI.Label(new Rect(0, 0, startRect.width, startRect.height), sFPS + " FPS", style); if (allowDrag) GUI.DragWindow(new Rect(0, 0, Screen.width, Screen.height)); } private void Awake() { // VSYNC 0: OFF // QualitySettings.vSyncCount = 0 // Application.targetFrameRate = 30; } }